Guide
Debugging Tool: The Must-Have Features
Table of Contents
Debugging is integral to software development, but becomes particularly challenging in complex, distributed systems. As systems scale and the list of components and dependencies grows, developers face a tedious process of sifting through myriad logs, metrics, and traces to uncover the relevant context for bugs.
Specialized debugging tools can greatly ease this burden by providing deep insights into system behavior and the circumstances surrounding the bug. Due to the complexity of modern systems, an effective debugging workflow must combine multiple tools to trace execution across components, monitor key metrics, and identify the root cause more efficiently. In this article, we explore the core features of debugging tools that help developers address the growing demands of contemporary system architectures.
Summary of key debugging tool features
Desired feature | Description |
---|---|
End-to-end traceability | Correlates logs and traces across microservices, supporting user-session or transaction tracing even in geographically distributed systems. |
Global state inspection | Collects metrics across all system components–including load balancers, message queues, and caches. Correlates these metrics with individual component states for comprehensive debugging. |
Centralized telemetry data | Consolidates session data and distributed traces, logs, and metrics in one place for seamless debugging. |
Contextualized debugging sessions | Provides high-level performance context and platform metrics across all those system resources to inform debugging efforts. |
Documentation | Consolidates vital information into a single location to break down knowledge silos and empower developers to debug with a comprehensive knowledge of the underlying system. |
The rest of the article explores these features in detail.
End-to-end traceability
Debugging distributed systems is challenging because the usual assumptions of local development no longer apply. Rather than running in a single process with shared memory and predictable execution order, services are spread across networks, execution is asynchronous, and state is fragmented across databases, caches, and queues. This introduces several challenges, such as:
- Clock synchronization – Synchronized timestamps across services are necessary for accurately stepping through execution flows. Distributed systems lack a single system clock, making it difficult to correlate logs and debug events precisely.
- Message passing complexity – In event-driven systems, messages traverse asynchronous queues, message brokers, or pub/sub systems. Debugging these workflows requires capturing message states and understanding event sequences.
- State management – Distributed debugging requires capturing snapshots of multiple states across different execution environments. Variables and execution states are not localized by default.
A modern debugging workflow should include tools that address these challenges. For example, distributed tracing tracks user request flows across services and provides visibility into both synchronous and asynchronous execution paths. Let’s take a closer look at how this is done.
Distributed tracing
Distributed tracing provides visibility into a request’s journey across multiple services. It tracks requests moving through different components and captures execution time, dependencies, and potential failure points. It also helps identify and diagnose protocol-level communication failures. Trace data for API requests, WebSocket connections, and other protocols helps detect timeouts, retries, dropped connections, and inconsistencies in request-response cycles. Teams can identify mismatched request formats, broken event flows, and errors caused by protocol mismatches across microservices.
Look for tools that provide the following types of distributed tracing capabilities.
Geo-distributed tracing using correlation and span IDs
Each request is assigned a correlation ID, which remains consistent across all services. Spans track specific operations, such as database queries or API calls, enabling developers to analyze performance at a granular level. For example, when an order processing system sends a payment request to a billing service, the same Correlation ID tracks the event through payment validation, transaction processing, and confirmation stages, even if these operations occur minutes or hours apart.
Asynchronous tracing
Asynchronous tracing tracks events that may be processed at different times, across different systems, or without a persistent connection.
Message tracing
Many distributed systems use message queues and event brokers (e.g., Kafka, RabbitMQ, AWS SQS) to facilitate asynchronous communication. To maintain trace continuity, your debugging tool should attach tracing metadata to messages. For example, the code block below demonstrates basic distributed tracing using Flask and OpenTelemetry:
from flask import Flask
import requests
from opentelemetry import trace
from opentelemetry.sdk.trace import TracerProvider
from opentelemetry.instrumentation.flask import FlaskInstrumentor
trace.set_tracer_provider(TracerProvider())
tracer = trace.get_tracer(__name__)
app = Flask(__name__)
FlaskInstrumentor().instrument_app(app)
@app.route("/process")
def process_request():
with tracer.start_as_current_span("process_request"):
# Trace external API call
with tracer.start_as_current_span("external_api_call"):
response = requests.get("https://api.example.com/data")
# Perform other tasks to trace executions
...
return "Request processed successfully"
if __name__ == "__main__":
app.run(debug=True)
The code uses the opentelemetry
library’s trace
functionality to trace requests as they flow through the application and capture information for downstream analysis. This data can then be exported to a backend like Jaeger or Prometheus to visualize and analyze the traces.
Visualizing tracing data
Your debugging tool should visualize tracing data so engineers can quickly diagnose anomalies and optimize system performance. Look for tools with the following visualization capabilities.
Capability | Explanation | Useful for |
---|---|---|
Flame graphs | Provide a hierarchical view of function execution, highlighting where most processing time is spent. | Profiling application performance and identifying slow operations. |
Gantt charts | Depict the sequence and duration of tasks across multiple services. | Analyzing task dependencies, execution order, and parallel processing. |
Percentile charts | Show the distribution of response times or resource usage. | Determining whether users are experiencing performance within acceptable thresholds or SLAs. |
Service maps | A graphical representation of service dependencies and request propagation | Showing how data flows between microservices, databases, and external APIs. |
Utilizing these visualization techniques, teams can accelerate root cause analysis and optimize system performance without sifting through raw trace logs.
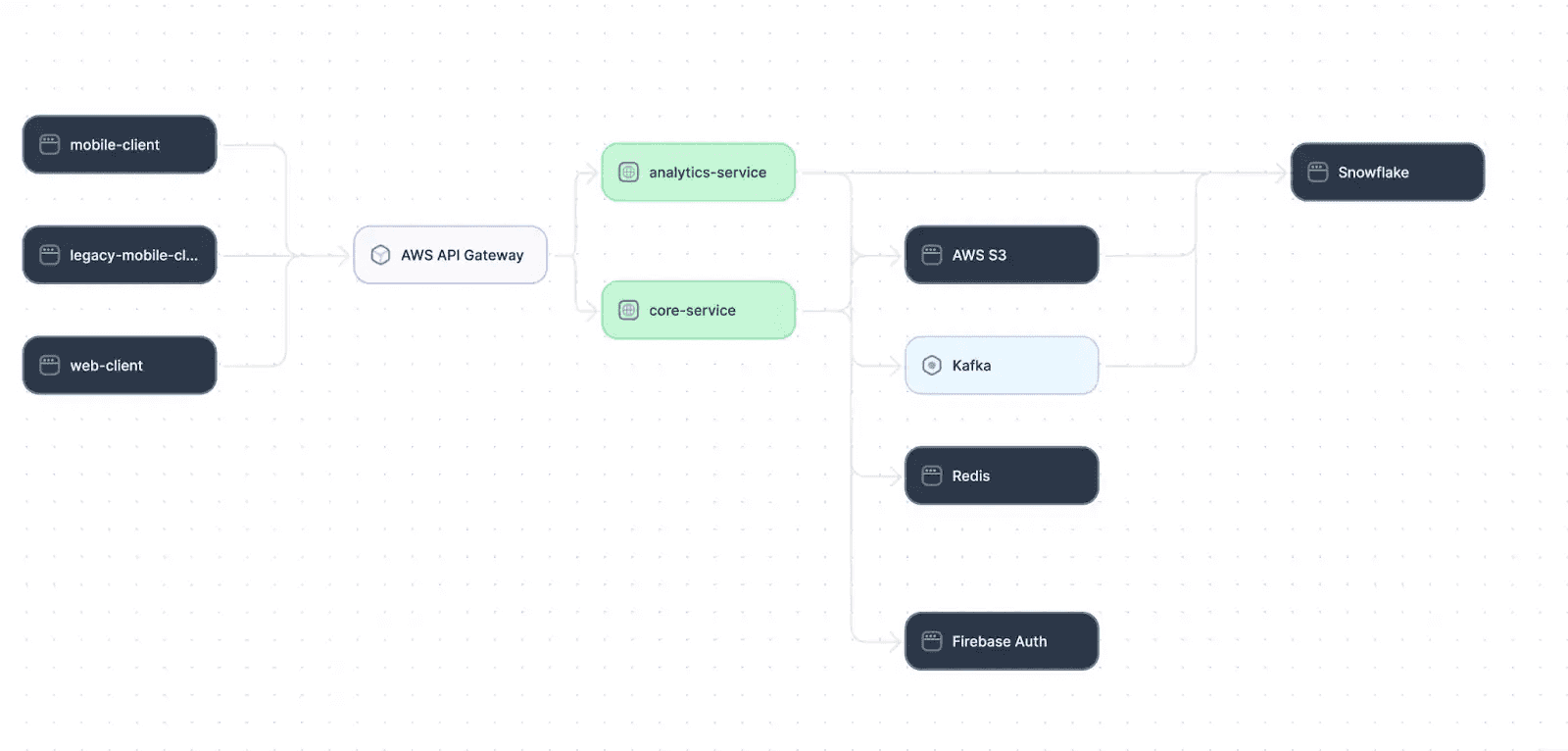
Example service map of a distributed system architecture
Tools for teams working on distributed systems
Learn moreGlobal state inspection
As mentioned previously, variables and execution states are not localized within distributed systems. As a result, effective debugging requires inspecting multiple states across different execution environments. Look for tools that allow developers to analyze both the overall system state and individual component health. Three important elements can assist in this process: metrics correlation, contextual enrichment, and real-time dashboards.
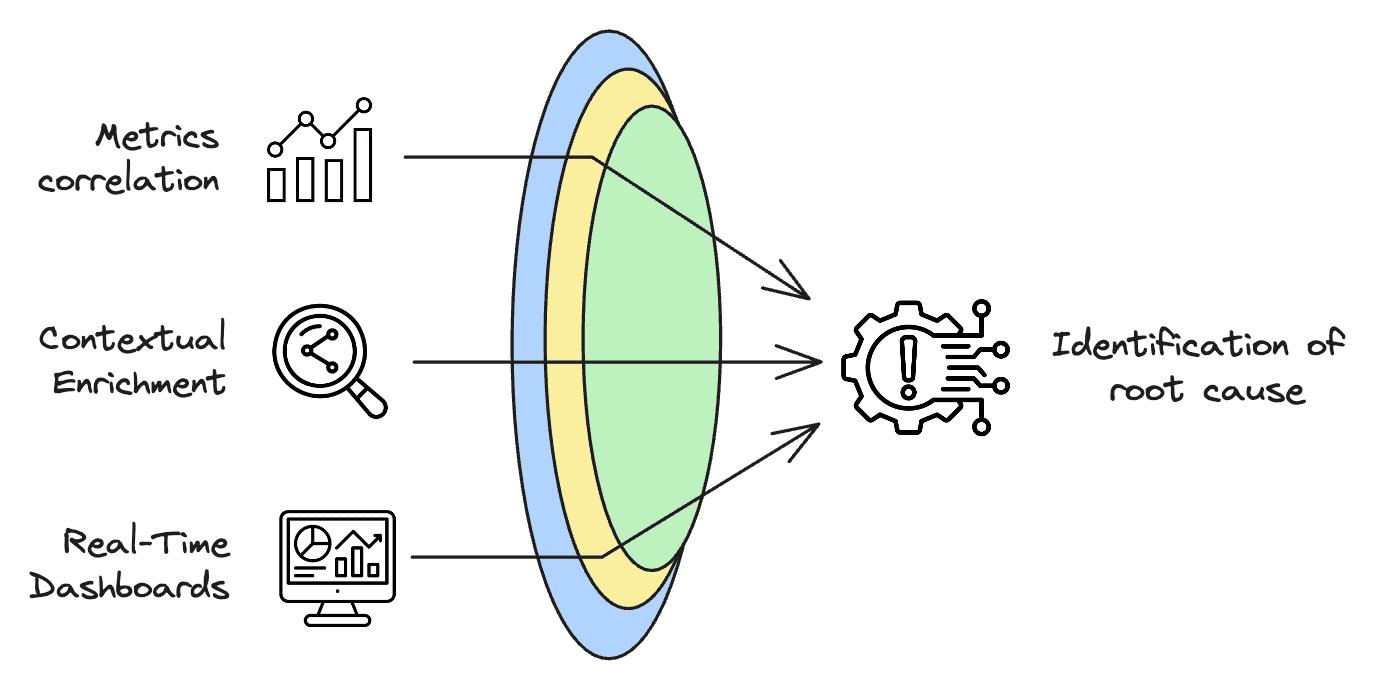
Illustration of global state inspection
Metrics correlation
Correlating performance metrics with traces and logs helps to understand how system-wide events impact application behavior. For example, teams can:
- Correlate load balancer metrics with backend service response times to reveal performance disparities and expose underlying infrastructure issues.
- Analyze load balancer metrics to detect misconfigurations or problems, such as failed health checks, security group restrictions, or network ACLs blocking traffic.
- Map infrastructure-level data–such as CPU and memory utilization–to service health to identify trends and proactively mitigate potential issues.
- Correlate application logs with specific user actions or requests to facilitate root cause analysis.
Including a debugging tool that aggregates these diverse insights in your workflow will help your team gain a comprehensive and data-driven understanding of system performance.
Contextual enrichment
Your debugging tool should augment raw data (like logs, metrics, and traces) with additional, relevant information about the system's state and environment when an event occurs.
Different types of contextual data provide different benefits. For example, including deployment versions with logs lets developers quickly see if an issue relates to a recent release. Configuration details offer insight into how settings affect runtime behavior. Metadata like geographic request origin or feature flag states allows granular debugging and can isolate problems to specific regions or features. Finally, the ability to capture frontend screens and correlate events (clicks, sign-in attempts, etc.) with the backend system’s distributed traces, metrics, and logs allows developers to gain a full picture of the bug without combing through APM data.
With these features, instead of just seeing an event’s outcome, developers gain insights into its root cause through information about the surrounding circumstances. In addition, because debugging is a collaborative process, the ability to quickly and easily share contextual data with other team members helps resolve bugs faster without lengthy email exchanges, message threads, or tickets. For an example of a tool that provides this functionality, check out Multiplayer’s Platform Debugger.
Real-time dashboards
Telemetry data collected from inspecting an application’s global state is most useful when visualized appropriately. Your debugging tool should provide real-time dashboards that do the following:
- Visualize your system and summarize information across different platforms, components, APIs, and dependencies.
- Automatically discover entities within your project and provide real-time information about discrepancies between your documentation and your live system.
- Auto-generate architecture diagrams based on the current state of your system.
- Visually highlight unusual patterns in metrics, logs, and traces, latency spikes, or error rate increases within the system.
- Dynamically map service dependencies and show how a problem in one component might cascade and affect other services. For example, a failing authentication service may affect a user profile service.
- Overlay past data on current metrics and trend lines to reveal performance changes after recent deployments or configuration updates.
Multiplayer’s System Dashboard is one example of a real-time dashboard. It integrates directly with your telemetry data and Multiplayer’s System Auto-Documentation, Platform Architecture Diagrams, and Platform Debugger to provide all the features above and capture insights from components, APIs, platforms, and dependencies. Doing so allows teams to gain a comprehensive, real-time view of system health and streamline debugging efforts.
Record session replays that include everything from frontend screens to deep platform traces, metrics, and logs
Start For FreeCentralized telemetry data
One of the biggest frustrations in debugging distributed systems is the inability to see the full execution path of a request. Frontend and backend logs often exist in silos. Issues arise when logs and traces are scattered across multiple services, making it difficult to pinpoint the root cause of failures. Ideally, your debugging tool should:
- Ensure that logs, traces, and metrics are preserved for complete issue reproduction.
- Highlight dependencies in microservices so you can identify how failures propagate across services.
- Unify logs, traces, and performance metrics into a coherent view to reduce time spent correlating scattered data.
Your debugging tool should merge logs from different services into a centralized, structured format to reduce the time spent piecing together events from isolated logs. That way, engineers can cross-reference execution traces with detailed log messages for deeper debugging insights.
It should also support log classification into different levels, such as info, debug, warn, and error, for faster incident resolution. Proper log-level categorization ensures more efficient troubleshooting and noise reduction in log analysis.
Let’s look at an example trace below.
{
"timestamp": "2025-03-05T14:23:45.678Z",
"level": "error",
"message": "Payment processing failed due to downstream service timeout",
"request_id": "abc123xyz",
"trace_id": "trc-456def789",
"service": "payment-service",
"operation": "charge_customer",
"dependencies": [
{
"service": "billing-service",
"status": "timeout",
"duration_ms": 5200
},
{
"service": "notification-service",
"status": "success",
"duration_ms": 150
}
],
"context": {
"customer_id": "cust-789",
"order_id": "ord-456",
"payment_method": "credit_card",
"retry_count": 2
},
"host": {
"ip": "10.1.2.3",
"region": "us-east-1"
},
"metrics": {
"response_time_ms": 5300,
"error_code": "GATEWAY_TIMEOUT"
}
}
This trace captures the full context of a failed payment processing operation. It identifies the cause (a timeout in the dependent billing service) and the customer and order IDs that precipitated the error. It also includes other key identifiers for reproduction. Data sources are consolidated into a structured format, allowing developers to investigate and troubleshoot the issue.
Contextualized debugging sessions
Even when logs are available, understanding how a failure fits into the broader system behavior is another challenge. Many debugging tools focus on only one part of the tech stack, such as frontend events, code introspection, or interactions between the browser and backend without tracking a request’s full path through the system. A tool that provides a complete picture of the bug via frontend and backend session data significantly enhances developers’ ability to troubleshoot. Key considerations include:
- Aligning debugging data with system behavior – Correlating service slowdowns, latency spikes, and bottlenecks with detailed execution traces helps developers understand "why" an issue occurred.
- Detecting environment-specific issues – Failures often emerge only under real-world conditions like high traffic, memory constraints, or resource exhaustion, making them hard to replicate in local environments.
- Synchronizing system metrics with debug sessions – Aligning real-time system metrics (e.g., CPU, memory, network, and disk I/O usage) with debugging timelines provides better execution context. It helps engineers pinpoint performance anomalies with debugging breakpoints.
Achieving these goals is often tedious and time-intensive. Developers may have to manually reproduce issues or comb through large quantities of APM data to find the relevant context for a problem. To add to these challenges, many teams lack a centralized location to store documentation, architecture diagrams, code repositories, and other essential information that allows developers to understand the underlying system comprehensively.
In short, debugging distributed systems often feels like piecing together a puzzle without a clear picture. Developers waste valuable time hunting down logs, manually correlating data, and documenting findings.
Tired of manually updating your system docs, APIs, and diagrams?
Learn How to AutomateTo alleviate this burden, your debugging tool should include:
- Session recording – Record all data needed to understand and recreate bugs to allow developers to see the full context behind an issue. These recordings should include frontend screens, backend traces, metrics, logs, and full request/response content and headers so that the team can understand everything that happened on the front and backend.
- Collaborative features – Effective debugging requires a team effort. The ability to share recordings of debugging sessions saves time and allows other team members to understand the bug comprehensively without long tickets, emails, or Slack conversations.
- User-recorded bugs – Some of the most frustrating bugs occur under circumstances the development team did not anticipate or is unable to reproduce. Allowing end-users to record deep session replays of unexpected behavior helps your team understand and resolve complex issues.
Tools like Multiplayer’s Platform Debugger include all the features above to help teams debug complex distributed systems. By providing centralized debugging insights and enabling better knowledge sharing, developers can avoid redundant troubleshooting and focus on fixing the real problems.
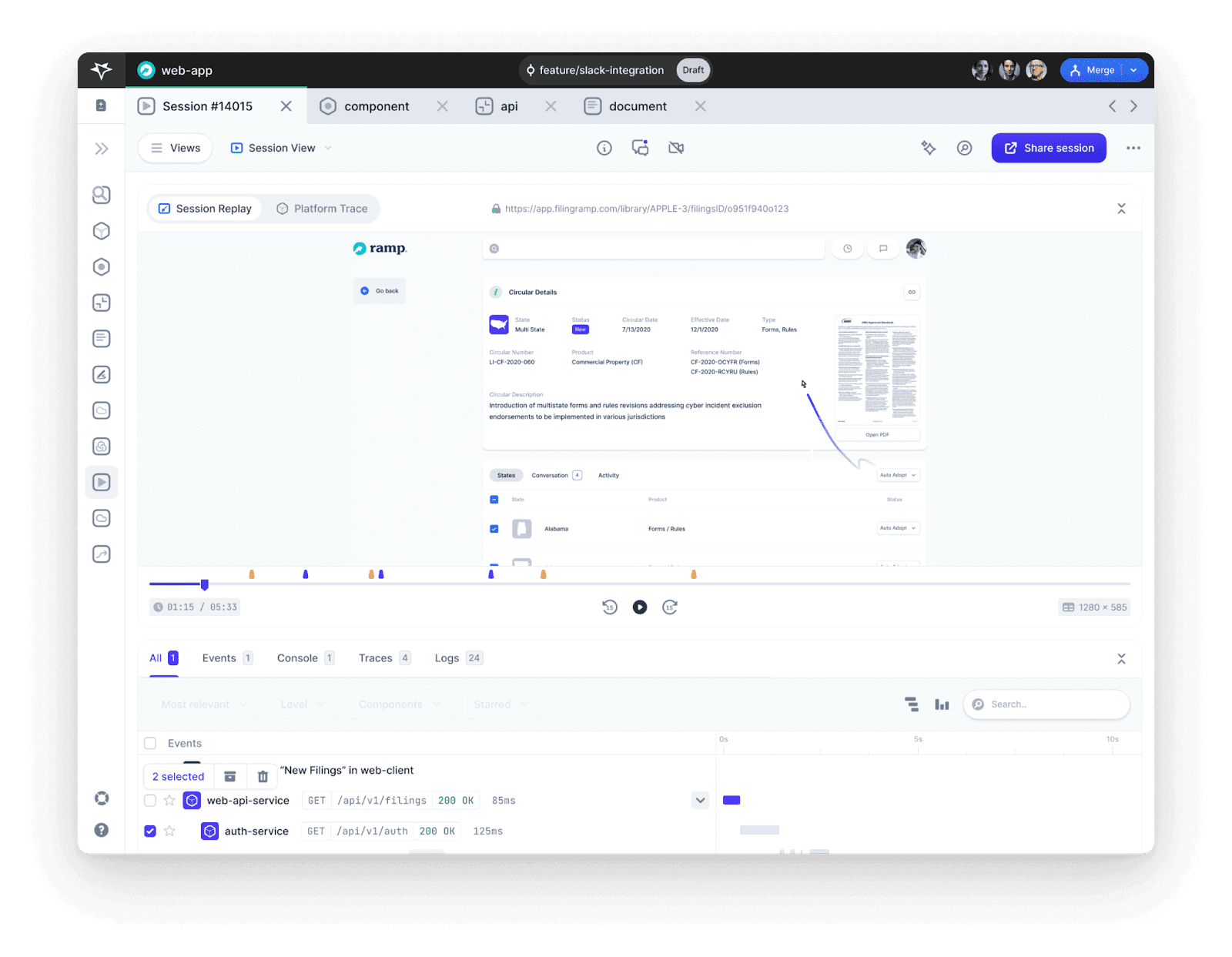
Multiplayer’s Platform Debugger
Documentation
Effective debugging in a complex, distributed environment hinges on a thorough understanding of how the system is built. Without up-to-date knowledge of the underlying architecture, developers are left either guessing at the source of issues or undertaking the time-consuming task of uncovering the system’s design layer by layer.
This often means navigating resources—documentation, architecture diagrams, decision records, APIs, repositories—scattered across Notion pages, Confluence wikis, Google Drive folders, Slack threads, internal portals, Git repositories, or even buried in personal notes and emails. Such fragmentation creates silos of knowledge, slows incident resolution, and often leads to duplicated effort or inconsistent understanding of the system’s current state.
To address these challenges, consider adopting a tool that combines documentation and live debugging in a single location. For example, Multiplayer’s Platform Notebooks provide live, executable API documentation that integrates directly with the Platform Debugger. In the context of a single Notebook, developers can construct and sequence API calls, inspect and validate responses, and capture full debugging session replays. This eliminates the need to dig through logs or switch between tools. It also ensures access to accurate, context-specific information and reduces reliance on stale or disconnected documentation.
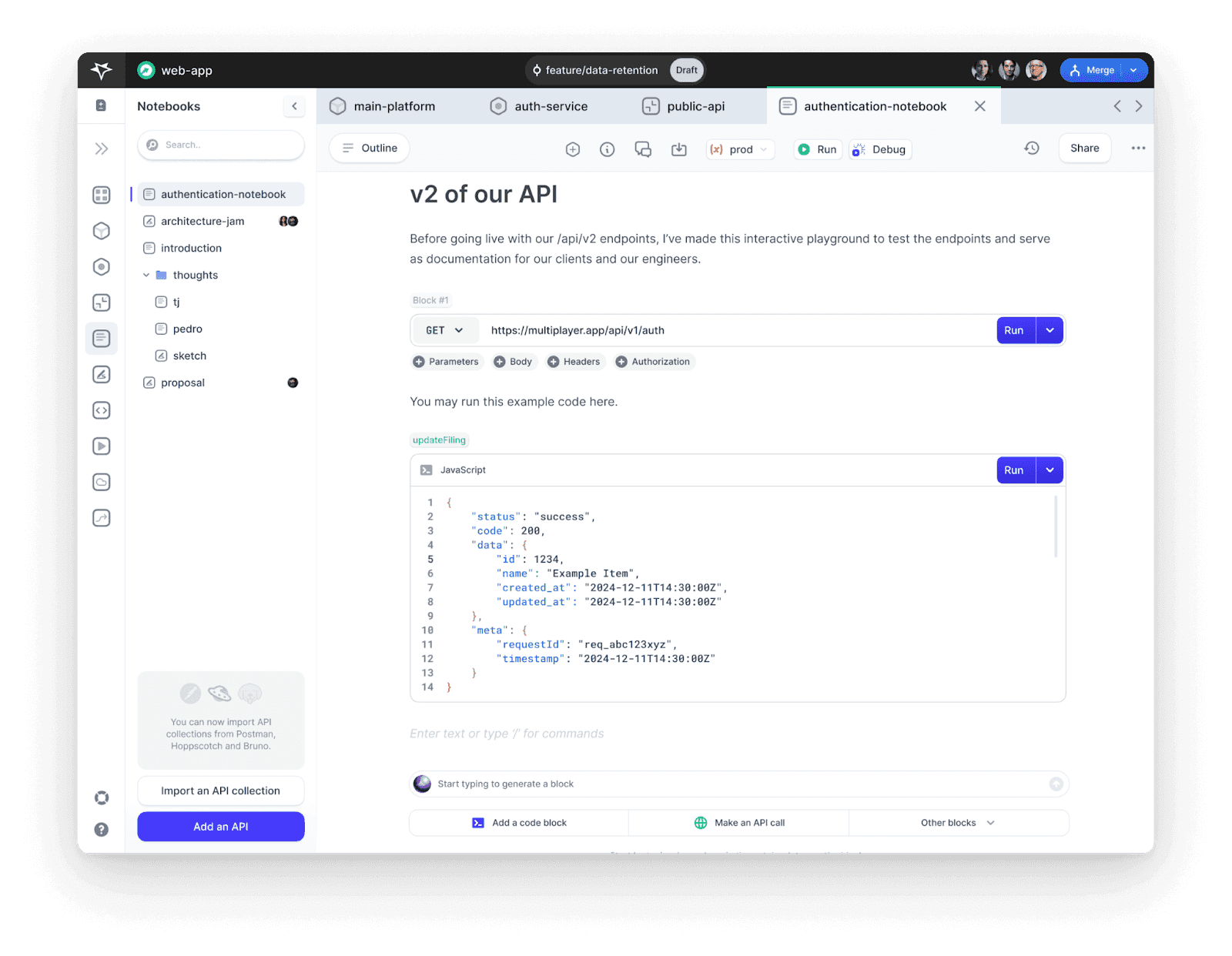
Multiplayer’s Platform Notebooks
Last thoughts
Effective debugging in distributed systems requires visibility, automation, and context-aware tools to streamline workflows and reduce inefficiencies. Traditional methods rely on manual data collection and fragmented logs, slowing issue resolution. Modern debugging tools address these challenges with cross-system breakpoints, global state inspection, end-to-end traceability, and automated contextualization. These tools enhance debugging efficiency by consolidating logs, traces, and performance metrics into a unified platform.
Multiplayer improves debugging with deep session replays, synchronized metrics, and auto-documentation, reducing manual effort and enabling faster issue resolution. Key benefits include seamless tracking of user requests, better correlation of system-wide states with component health, and collaborative debugging workflows that minimize downtime. By adopting these advanced capabilities, teams can enhance developer productivity, improve system reliability, and maintain high-performing distributed architectures.